- Python Msvcrt Install
- Python Msvcrt For Mac Catalina
- Python Msvcrt For Machine Learning
- Install Msvcrt
- Python Msvcrt Not Working
- Python Msvcrt For Mac Operating System
Extended for MacOS. A few modifications make this work for the mac with Carbon, too: a modification to the Getch to try one more import, a modification to the Unix init, and the inclusion of the instructions for the MacOS with Carbon support. Sheila King On Sat, 25 Aug 2001 16:16:39 -0400 (EDT), Ignacio Vazquez-Abrams wrote in comp.lang.python in article::I have no experience with msvcrt, but I'm guessing that it might be that:msvcrt.locking requires the descriptor to be the same when unlocking as when:locking. I want to use the msvcrt module in python, but I can't make it work. I am on windows 10, have several 'active keyboards' (I can change it from azerty to qwerty and stuff) and did not do/download anything except Python3 itself (I'm saying that because maybe there are some requirements or manipulations to do that I don't know about). The getch module does single-char input by providing wrappers for the conio.h library functions getch (gets a character from user input, no output - this is useful for password input) and getche (also outputs to the screen), if conio.h does not exist, it uses a stub-library using termios.h and other headers to emulate this behaviour.
Released:
Does single char input, like C getch/getche
Project description
The getch module does single-char input by providing wrappers for the conio.h library functions getch() (gets a character from user input, no output - this is useful for password input) and getche() (also outputs to the screen), if conio.h does not exist, it uses a stub-library using termios.h and other headers to emulate this behaviour:
Hint: On Windows, you can use:
as a standard library alternative to this module
Release historyRelease notifications | RSS feed
Download files
Download the file for your platform. If you're not sure which to choose, learn more about installing packages.
Filename, size | File type | Python version | Upload date | Hashes |
---|---|---|---|---|
Filename, size getch-1.0-python2.tar.gz (1.2 kB) | File type Source | Python version None | Upload date | Hashes |
Filename, size getch-1.0.tar.gz (1.3 kB) | File type Source | Python version None | Upload date | Hashes |
Hashes for getch-1.0-python2.tar.gz
Algorithm | Hash digest |
---|---|
SHA256 | be451438f7a2b389f96753aea39b6ed2540a390f1b9a12badcbc110cf9a5ce7f |
MD5 | 586ea0f1f16aa094ff6a30736ba03c50 |
BLAKE2-256 | 56f7cde35f44d267df7122005c40f1a15cf5e3c60ffc83a2ab00d11d99e9d8c4 |
Hashes for getch-1.0.tar.gz
Algorithm | Hash digest |
---|---|
SHA256 | a6c22717c10051ce65b8fb7bddb171af705b1175e694a73be956990f6089d8b1 |
MD5 | 57519f64807285bdfff8e7b62844d3ef |
BLAKE2-256 | cca4c696c05e0ff9d05b1886cb0210101083db7d330ff964a6d7cd98ad2b2064 |
Released:
A simple, cross-platform module for mouse and keyboard control

Project description
A module for cross-platform control of the mouse and keyboard in python that is
simple to use.
Mouse control should work on Windows, Mac, and X11 (most Linux systems).
Scrolling is implemented, but users should be aware that variations may
exist between platforms and applications.
Keyboard control works on X11(linux) and Windows systems. Mac control is a work
in progress.
Dependencies
------------
Depending on your platform, you will need the following python modules for
PyUserInput to function:
* Linux - Xlib
* Mac - Quartz, AppKit
* Windows - pywin32, pyHook
How to get started
------------------
After installing PyUserInput, you should have pymouse and pykeyboard modules in
your python path. Let's make a mouse and keyboard object:
```python
from pymouse import PyMouse
from pykeyboard import PyKeyboard
m = PyMouse()
k = PyKeyboard()
```
Here's an example of clicking the center of the screen and typing 'Hello, World!':
```python
x_dim, y_dim = m.screen_size()
m.click(x_dim/2, y_dim/2, 1)
k.type_string('Hello, World!')
```
PyKeyboard allows for a range of ways for sending keystrokes:
```python
# pressing a key
k.press_key('H')
# which you then follow with a release of the key
k.release_key('H')
# or you can 'tap' a key which does both
k.tap_key('e')
# note that that tap_key does support a way of repeating keystrokes with a interval time between each
k.tap_key('l',n=2,interval=5)
# and you can send a string if needed too
k.type_string('o World!')
```
and it supports a wide range of special keys:
```python
#Create an Alt+Tab combo
k.press_key(k.alt_key)
k.tap_key(k.tab_key)
k.release_key(k.alt_key)
k.tap_key(k.function_keys[5]) # Tap F5
k.tap_key(k.numpad_keys['Home']) # Tap 'Home' on the numpad
k.tap_key(k.numpad_keys[5], n=3) # Tap 5 on the numpad, thrice
```
Note you can also send multiple keystrokes together (e.g. when accessing a keyboard shortcut) using the press_keys method:
```python
# Mac example
k.press_keys(['Command','shift','3'])
# Windows example
k.press_keys([k.windows_l_key,'d'])
```
Consistency between platforms is a big challenge; Please look at the source for the operating system that you are using to help understand the format of the keys that you would need to send. For example:
```python
# Windows
k.tap_key(k.alt_key)
# Mac
k.tap_key('Alternate')
```
I'd like to make a special note about using PyMouseEvent and PyKeyboardEvent.
These objects are a framework for listening for mouse and keyboard input; they
don't do anything besides listen until you subclass them. I'm still formalizing
PyKeyboardEvent, so here's an example of subclassing PyMouseEvent:
```python
from pymouse import PyMouseEvent
def fibo():
a = 0
yield a
b = 1
yield b
while True:
a, b = b, a+b
yield b
class Clickonacci(PyMouseEvent):
def __init__(self):
PyMouseEvent.__init__(self)
self.fibo = fibo()
def click(self, x, y, button, press):
''Print Fibonacci numbers when the left click is pressed.''
if button 1:
if press:
print(self.fibo.next())
else: # Exit if any other mouse button used
self.stop()
C = Clickonacci()
C.run()
```
Intended Functionality of Capturing in PyUserInput
--------------------------------------------------
For PyMouseEvent classes, the variables 'capture' and 'capture_move' may be
passed during instantiation. If `capture=True` is passed, the intended result
is that all mouse button input will go to your program and nowhere else. The
same is true for `capture_move=True` except it deals with mouse pointer motion
instead of the buttons. Both may be set simultaneously, and serve to prevent
events from propagating further. If you notice any bugs with this behavior,
please bring it to our attention.
A Short Todo List
-----------------
These are a few things I am considering for future development in
PyUserInput:
* Ensuring that PyMouse capturing works for all platforms
* Implement PyKeyboard capturing (add PyKeyboardEvent for Mac as well)
* PyMouse dynamic delta scrolling (available in Mac and Windows, hard to standardize)
* Make friends with more Mac developers, testing help is needed...
Many thanks to
--------------
[Pepijn de Vos](https://github.com/pepijndevos) - For making
[PyMouse](https://github.com/pepijndevos/PyMouse) and allowing me to modify
and distribute it along with PyKeyboard.
[Jack Grigg](https://github.com/pythonian4000) - For contributions to
cross-platform scrolling in PyMouse.
Release historyRelease notifications | RSS feed
0.1.11
0.1.10
0.1.9
0.1.8
0.1.7
0.1.6
Python Msvcrt Install
0.1.5
0.1.4
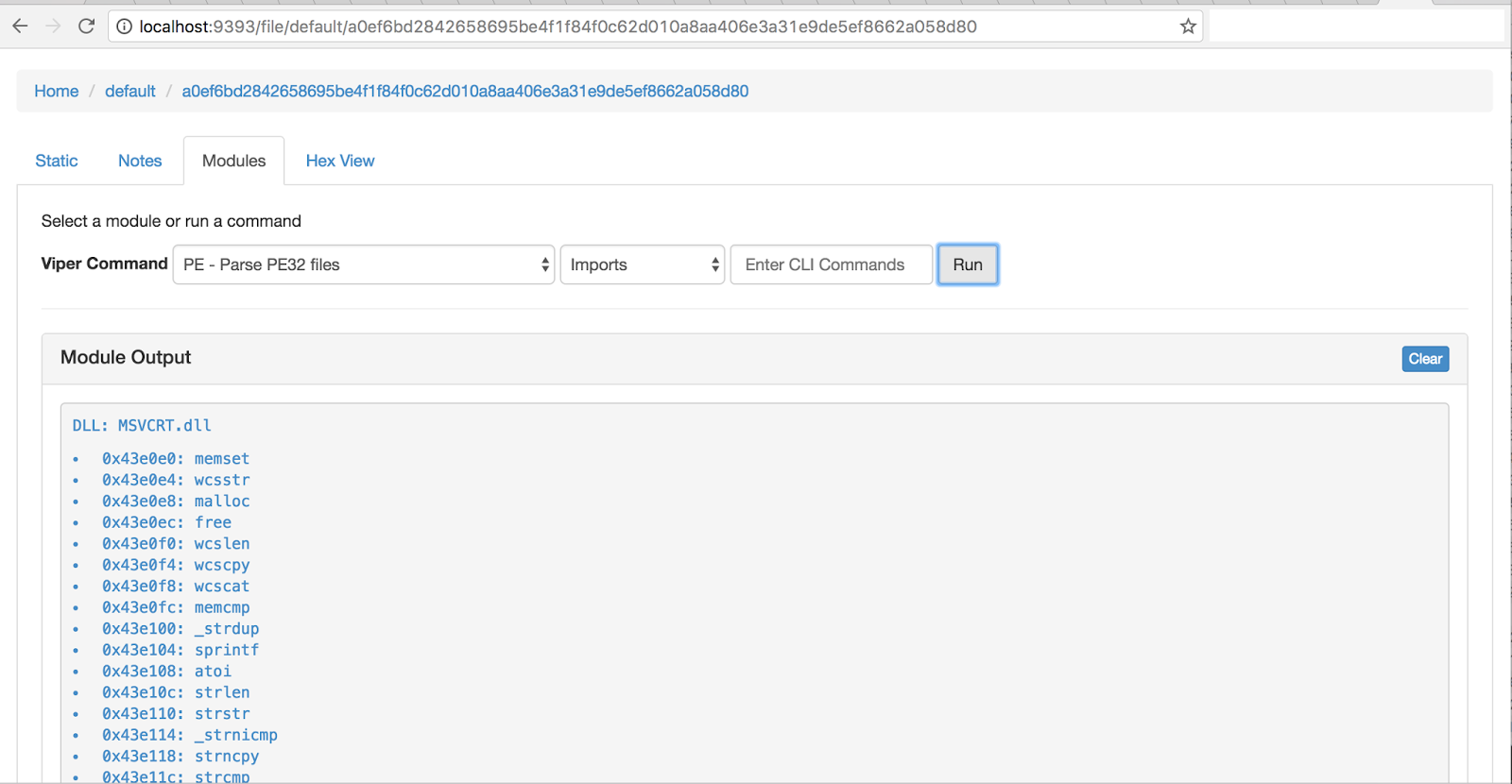
0.1.3
0.1.2
0.1.1
0.1
Python Msvcrt For Mac Catalina
Download files
Download the file for your platform. If you're not sure which to choose, learn more about installing packages.
Filename, size | File type | Python version | Upload date | Hashes |
---|---|---|---|---|
Filename, size PyUserInput-0.1.11-py2-none-any.whl (40.0 kB) | File type Wheel | Python version 2.7 | Upload date | Hashes |
Filename, size PyUserInput-0.1.11.tar.gz (28.7 kB) | File type Source | Python version None | Upload date | Hashes |
Hashes for PyUserInput-0.1.11-py2-none-any.whl
Python Msvcrt For Machine Learning
Algorithm | Hash digest |
---|---|
SHA256 | 61d9c0e81331b02efa5c62c6fb9416675ae79fbd00a3e12eaa8274b103446857 |
MD5 | 061b539ff3783b72e42e37915c59a8ef |
BLAKE2-256 | fca7ed646570dcbef763da66e27e6812dc6723c42e81d0b72a8ace184c40dc89 |
Install Msvcrt
ClosePython Msvcrt Not Working
Hashes for PyUserInput-0.1.11.tar.gz
Python Msvcrt For Mac Operating System
Algorithm | Hash digest |
---|---|
SHA256 | 006b5ed06cf740eeeb2c7221e1cb8dc5ae35f16267e41262bfb0e7cfdca7fb2b |
MD5 | 2095c70da5e48c2954588470444a4937 |
BLAKE2-256 | d00917fe0b16c7eeb52d6c14e904596ddde82503aeee268330120b595bf22d7b |
